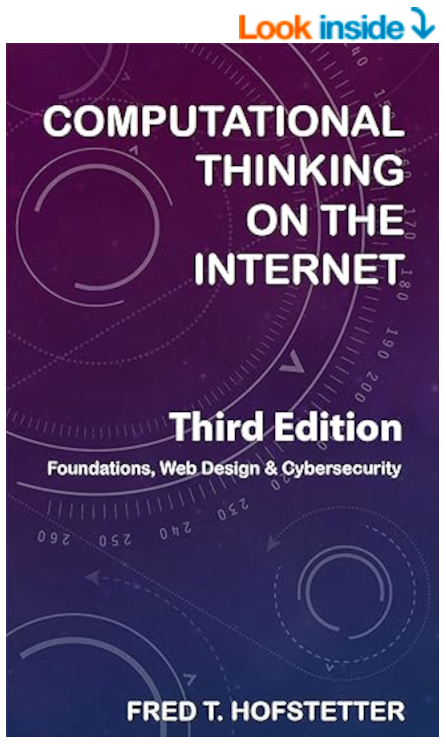
Computational Thinking on the Internet
Chapter 7: JavaScript Programming and the DOM
After completing Chapter 7, you will know how to:
- List the primary scripting languages, understand the role of JavaScript, and write a script that uses variables to display dynamic messages onscreen.
- Understand the purpose of the Document Object Model (DOM), describe the most popular JavaScript DOM objects, and use dot notation to access those objects in a script.
- Explain how objects have methods and properties that can be called upon to do things when events happen as the user interacts with your Web page.
- Program the JavaScript Date object and manipulate its methods and properties.
- Understand the concept of a cookie and write a script to maintain the user’s state by setting and reading the values of cookies as the user interacts with your site.
- Debug a script to fix problems if the script is not working correctly.
End of Chapter Labs
Lab Project 7.1:Inspecting Your Cookies
If you have never looked around your computer to see what cookies are stored there, you will be amazed by what this lab project will turn up. Remember that there are two kinds of cookies: (1) per-session cookies that are stored in RAM and evaporate when the user closes the browser windows, and (2) persistent cookies that survive from session to session. Persistent cookies endure even when the user reboots or powers off the computer. The reason why persistent cookies stick around is because the browser stores them in the computer's local storage. To inspect the cookies that the browser is storing locally on your computer, follow these steps:
Windows
- Get the Windows File Explorer running and select the root of the drive you want to search.
- In the search field, type the word cookie and press Enter.
- Wait while your computer looks for filenames containing the word cookie. One by one, your cookie files will begin to appear in the search window.
- Look for a folder called cookies. Right-click it and choose the option to enter that folder. Here you will probably find many more cookie files.
- To inspect a cookie file, right-click its filename to bring up the quick menu, choose Open, and choose the option to open the file with the Notepad. Many cookies are encrypted, so do not be disappointed if you cannot decipher the contents of these files.
- Reflect on how servers all over the Internet, including commercial websites, are using your computer’s persistent memory as a storage medium.
Macintosh
- Get Safari running.
- From the Safari menu, select Preferences.
- In the Safari preferences window, choose Privacy.
- Use the option to Manage Website Data and peruse all the sites that are storing information on your computer.
- To inspect the contents of a specific cookie, use Safari to go to its website.
- Pull down Safari’s Develop menu and choose the Web Inspector.
- Choose the Storage tab and click Cookies. Many cookies are encrypted, so do not be disappointed if you cannot decipher the contents of these files.
- Reflect on how servers all over the Internet, including commercial websites, are using your computer’s persistent memory as a storage medium.
If your instructor asked you to hand in this Cookies lab, use your word processor to write an essay in which you report on the cookies you found and reflect on the purpose they serve. As you know from viewing this YouTube video earlier in this chapter, the Internet is stateless by design. Every time you interact with the Internet, it hangs up on you by closing the socket through which you connected. Cookies were invented to provide a way for applications to maintain state by keeping records either in per-session cookies that evaporate when the user closes the browser, or in persistent cookies that remain on your computer from session to session. There is no way for you to see the per-session cookies that evaporate. In this lab, you have seen the cookies that persist from session to session. In your essay, describe the cookies you found and write about the purpose you think they serve. Did any of the cookies keep data that surprised you? Did you find any of the cookies to be invasive of your privacy? Were some of them encrypted such that you were unable to see what they contained? If you do not like having cookies on your computer, you can use your browser settings to turn cookies off. Doing so, however, will make many of the Internet’s web services stop working on your computer. Did you find anything in your cookies that makes you wish you could turn them off? When you hand in this essay, make sure you put your name at the top of the page, then copy it onto a disk or follow the other instructions you may have been given for submitting this assignment.
Lab Project 7.2: Dynamic HTML
Dynamic HTML is a term invented by Microsoft to refer to the animated Web pages you can create by using the DOM to combine HTML with style sheets and scripts that bring Web pages to life. Some people get confused by the term Dynamic HTML, because they think it refers to some kind of a product. Dynamic HTML is not a product; rather, it is a concept. Whenever you create dynamic effects onscreen by manipulating objects in the DOM, you are doing Dynamic HTML. In this lab, you learn how Dynamic HTML works by using JavaScript to modify the x,y location of an image onscreen. By using a timer to change the location periodically, you can make the image move across the screen. To create such an animation, follow these steps:
- Pull down your Code editor’s File menu and choose New to create a new file. Then use the File menu to Save this new file under the filename dynamic.html.
- Into this new file, enter the following code:
<!DOCTYPE html>
<html>
<head>
<title>Dynamic HTML Example</title>
<script language="JavaScript">
var timerID;
var x = 0;
var y = 0;
function BeginAnimation()
{
timerID = window.setInterval("ContinueAnimation()",50);
}
function ContinueAnimation()
{
x += 10;
MyPhoto.style.left = x + 'px';
y += 4;
MyPhoto.style.top = y + 'px';
if (x>200)
{
window.clearInterval(timerID);
}
}
</script>
</head>
<body onload="BeginAnimation()" marginheight="0" topmargin="0" leftmargin="0">
<img id="MyPhoto" style="position:absolute; top:0px; left:0px" src="photo.jpg">
</body>
</html> - In the third last line of this code sample, where the image name is photo.jpg, type the name of the image you want to animate.
- Pull down the File menu and choose Save. Then open the dynamic.html file with your browser. You will see the image move around the screen as the script alters the x,y values that position the image onscreen.
Dynamic HTML Code Analysis
In order to create an animation with Dynamic HTML, you need to latch on to an event that can get the animation started. The dynamic.html script does this in the <body> tag via the onload event, which is one of the intrinsic events identified earlier in this chapter in Table 7.3. Notice how the <body> start tag is programmed to fire the BeginAnimation() function when the page loads:
<body onload="BeginAnimation()">
The BeginAnimation() function is very brief. It calls upon the setInterval() method of the JavaScript window object to set a timer that will go off after 50 milliseconds and fire the ContinueAnimation() function. You can make the animation happen faster or slower by changing the value 50 in the code below:
function BeginAnimation()
{
timerID = window.setInterval("ContinueAnimation()",50);
}
After you get an animation started, you need to keep it going. The ContinueAnimation() function does that by computing the image’s next position and setting another timer. This process continues until the image moves past the point at which the IF statement stops the animation by calling upon the clearInterval() method to stop the timer from going off any more:
function ContinueAnimation()
{
x += 10;
MyPhoto.style.left = x + 'px';
y += 4;
MyPhoto.style.top = y + 'px';
if (x>200)
{
window.clearInterval(timerID);
}
}
In order to keep the coding straightforward, this example moved the image along a straight line. If you know your math, however, there is no limit to the patterns of movement you can create onscreen. In the ContinueAnimation function shown here, MyPhoto.style.left controls how far over the image will appear, and MyPhoto.style.top is how far down the picture will move.
Submitting the Dynamic HTML Lab
If your instructor asked you to submit this lab, do so by submitting the HTML file in which you created your Dynamic HTML example. In your script, you should use comment statements to document what your code is doing. In JavaScript, you create a comment statement by typing // followed by the comment you want to make. Here is an example of a comment statement explaining what the code is doing:
//Stop the timer when the animation has moved 200 pixels
if (MyPhoto.style.left>200)
{
window.clearInterval(timerID);
}
Lab Project 7.3: Blockly, App Lab, and Your Hour of Code
In this computational thinking module, you will accomplish the following learning objectives:
- Create an app that you can distribute via Facebook or Twitter or text to any smartphone.
- Learn how to think computationally by strategizing how to decompose problems, design algorithms, manipulate data, and use abstractions as you add features to your app.
- Experience how computer scientists use named procedures to solve not only the task at hand but also other tasks to which these procedures may apply in the future.
- Reflect on how this process of building capability through the development of shared libraries of named procedures empowers people, working groups, and entire disciplines to progress and move their fields forward.
If these learning objectives sound lofty and foreboding, worry not, because the learning activities you will experience in this chapter are designed in such a way that you will enjoy building your own app and reflecting on what it means to think computationally about how the world works. Follow this link for the complete text of the lab.