Programming Languages
The central UNIX systems (Strauss and Mahler) at the University of Delaware offers a variety of programming languages. If you are not familiar with computer programming, select "General information--Creating and Running a Program" before you try to write your program. If you want information on how to use a particular language on Strauss and Mahler, select the appropriate heading below to go directly to the section on that language.
Programming Languages
Following are some of the programming languages available on the central UNIX
systems, Strauss and Mahler:
AcuCOBOL BinProlog Workshop C
GNU C WorkShop C++ GNU C++
Liquid Common Lisp Scheme Workshop Fortran 77
WorkShop Fortran 90/95 GNU Pascal
On-line "man" pages as well as
Sun's AnswerBook
provide information on most of the above languages. Table 1.1 lists the appropriate "man" command for each language, shows the file suffix to use, and the command to compile the program. This document gives tips for using the C, Pascal, Fortran 77, and Scheme languages on Strauss and Mahler.
Note: If you have trouble accessing the compilers and man pages, you may not be using the current prototype setup files (.cshrc, .login, .localalias, .localenv). To copy these files to your account, type
unset noglob; ~consult/proto/setup
Then log out and log in again. Any setup files you already had on your account will be saved under new names (the filename with a date appended to it).
Table 1.1 Programming language summary
Language Suffix Compile Help
AcuCOBOL .cbl ccbl ccbl -h
BinProlog .bp bp man bp
C (WorkShop) .c cc man cc
C (Gnu) .c gcc man gcc
C++ (WorkShop) .cc CC man CC
C++ (Gnu) .cc g++ man g++
Fortran 77 (WorkShop) .f f77 man f77
LISP (Liquid Common) .lisp lisp ---
(to load)
Fortran 90 (WorkShop) .f90 f90 man f90
Pascal (GNU) .p pc man pc
Scheme .s scm man scm
(See also DrScheme)
If you are a beginner, you should use the flow chart in Figure 1.1 and the accompanying notes as a guide in developing your program. You will learn only basic techniques in this section. Advanced topics, such as the use of debuggers and data files, can be found in the appropriate language reference manuals.
Table 1.1 lists information you will need to create and execute your program. Refer to it as you read through this part of the chapter, paying particular attention to the information that pertains to the language you are using.
Before you start writing a program, you must understand the problem and what is required to solve it. Your own flow chart is useful at this point so that you can appreciate the steps that will be involved in that solution, the problems that may arise, and what action has to be taken. You should work through your flow chart with some sample data to be sure you have not missed some vital computation or checks. When you are satisfied with your flow chart, you can begin to write your program. This process involves translating your flow chart into a series of statements the computer can understand.
Figure 1.1 Writing a Program: A General Flow Chart
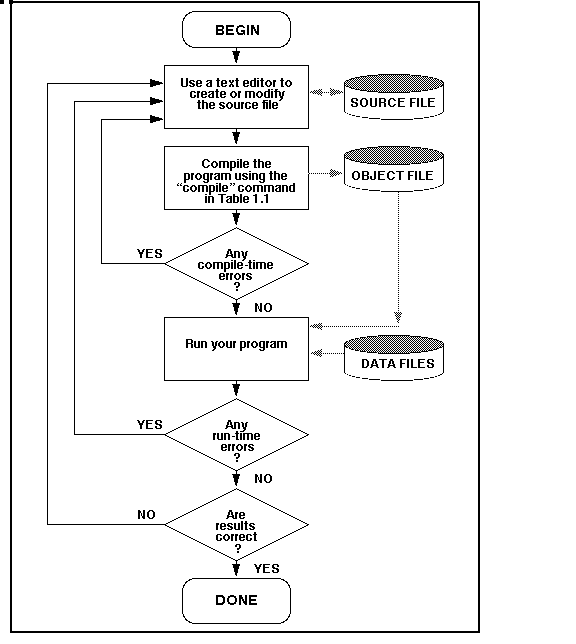
A source file is the file that contains your program. Use a text editor (pico or vi) to type your program into the file or to edit it. The file may have any name, but it must have an extension that corresponds to the programming language you are using (see Table 1.1).
After you have typed your program into a source file using a text editor, save the program with the appropriate command for the editor you're using. Next compile the program using the compiler command in the "Compile" column of Table 1.1 that corresponds to the language you are using. For example, to compile a Fortran program "prog1.f", you would type
f77 prog1.f
and press the RETURN key. The compiler performs two functions:
- It checks the syntax of each statement in your program.
- It generates an object file that contains the machine language version of your program; this is the version that is actually run by the computer.
Errors the compiler finds in your source code are called compile-time errors or syntax errors. You need to determine the nature of the errors and where they occur in your program before you can correct them. When you have done this, use a text editor to make the necessary changes to your file; then recompile your program.
Once your program is free of syntax errors, you can execute (run) it. However, the program may not produce the results you intended because of errors in logic. Again, you must determine the nature of the errors, use a text editor to correct them, and recompile your program. Some typical causes of run-time errors are
- Division by zero (divide check). Your program contains an expression of the form X/Y where Y has a value of zero.
- Data format error. Reading a character into a numeric variable will cause this error. Check that the sequence of read statements matches the data being read.
- Read past the end-of-file. You may be attempting to read data from an empty file, or you may not be properly checking for the end-of-file condition.
- Array subscript out of range (high-bound or low-bound checking error). For example, if you define an array with subscript values of 1,2,...,10 and you have a subscript value of 0 or 11, you will get this error. Check that subscripts are being computed properly.
- Infinite loops. Check whether you have omitted the read statement from the body of the loop. Did you forget to increment the loop counter? Are you branching to the wrong place in your program?
- Get in the habit of "echoing" input data and intermediate results. Echoing means inserting extra output statements to print the values of program variables so you can more easily follow the execution of your program. For example, if you have an array subscript problem, echo the values of the subscripts as they are computed; if you have a data problem, echo the data as it is being read in; or if you have a problem with a subprogram or procedure, echo the arguments. Echoing is a simple but useful debugging technique that can help you get to the root of the problem.
- If the program printed the wrong answers, check
the output statement that produced them. Are you specifying the correct variables? If there is nothing wrong here, then work
back from the output statement. If the values you are printing are ones that your program read, you should insert an output statement after the statement that read those values in. Maybe your input statement is wrong, or perhaps you typed the values incorrectly.
If the program computes the values, insert output statements immediately after the computation is done. Remember, the value of a variable is changed if the variable name is on the left-hand side of an assignment statement, in an input statement, the control variable of a loop statement (e.g., "do" in Fortran 77, "for" in Pascal), or a parameter that is changed by a procedure or subroutine. (Lost? Go back to your textbook or language manual!)
- If you are getting unexpected results--for example, your final set of results is printing before anything else--then control of the program is not being transferred as you thought it would be. Statements like "goto" and "if" transfer control. Insert an output statement that writes out the values of the variables used in the conditional statement before that statement is obeyed.
- If your program has not printed anything, you know it has not encountered a single output statement. Look at your program and find the output statement that you expected to be encountered first. Because nothing has printed, you know the program did not get that far. Go to the start of your program and look for any statements that might have transferred control of the program either back to a point near the beginning of the program or to a point beyond the output statement.
- Often there is more than one way to correct an error. You must decide which is best.
Following are suggestions for creating and running programs in C on UNIX. If you are a beginner or unfamiliar with UNIX, you should read the section "Writing Your Program." To create and run a C program, follow these steps
- Create your program source file using a text editor. The source file can have any filename that ends with ".c". For example, you could create the following program called "test.c":
#include<stdio.h>
main ()
{
printf("Hello, World!\n");
}
- After you have saved your program with the editor, compile it using the "CC" compiler. "CC" compiles a C program and, if it is free of syntax errors, creates an executable file (object program) in your current directory.
- You can compile your program in two ways:
- To run (execute) the program, type "a.out" or "test.cout" from the C Shell, depending upon which form of the command you used to compile your program.
Sometimes a single error in a program can cause further errors. You will not be able to execute the program correctly until these errors are fixed. Don't forget to recompile ("CC") your program after making changes. Otherwise, you'll be running an old and incorrect version when you type "a.out" or programname.cout (the filename you gave after the -o option).
Programs in C usually require a library of routines. The above example used the library "stdio" for the standard I/O routines. There are usually two things you need to do for each library your program requires.
- Include the appropriate header file using the "#include" statement.
- Pass the "-llibrary" option to the loader.
The "CC" command will pass trailing options to the loader, so you must put the "-llibrary" option as the last option of the "CC" command.
The standard I/O routines are a special case because they do not require any options. As a typical example, consider the case of the mathematical functions. If your program calls any mathematical functions such as "sqrt" or trigonometric functions, you need the math library. Put the line
#include <math.h>
in your source file and compile it with the command:
cc test.c -lm
Following are suggestions for creating and running Pascal programs on UNIX. If you are a beginner or unfamiliar with UNIX, you should read the section "Writing Your Program." To create and run a Pascal program, follow these steps
- Create your program source file using a text editor (vi or pico). The source file can have any filename that ends with ".p". For example, you could create the following program called "test.p":
Program hello;
Begin
Writeln('Hello, World!');
End.
- After you have saved your program with the text editor, compile it using the "pc" compiler. "pc" compiles a Pascal program and, if it is free of syntax errors, creates an executable file (object program) in your current directory.
- You can compile your program in two ways. In each case, the -L option is used to ignore uppercase characters.
- If you type
pc -L test.p
Pascal will create "a.out" as the executable file.
- If you type
pc -L -o test.pout test.p
Pascal will create "test.pout" as the executable file.
- To run (execute) the program, type "a.out" or "test.pout" from the C Shell, depending upon which form of the command you used to compile your program.
Sometimes a single error in a program can cause further errors. You will not be able to execute the program correctly until these errors are fixed. Don't forget to recompile ("pc") your program after making changes. Otherwise, you'll be running an old and incorrect version when you type "a.out" or programname.pout (the filename you gave after the -o option).
Following are suggestions for creating and running Fortran 77 programs on UNIX. If you are a beginner or unfamiliar with UNIX, you should read the section "Writing Your Program." To create and run a Fortran 77 program, follow these steps
- Create your program source file using a text editor (pico or vi). The source file can have any filename that ends with ".f". For example, you could create the following program called "test.f". Make sure to use a tab at the beginning of each line:
write (*,*) 'Hello, World!'
end
- After you have saved your program with the text editor, compile it using the "f77" compiler. "f77" compiles a Fortran 77 program and, if it is free of syntax errors, creates an executable file (object program) in your current directory.
- You can compile your program in two ways:
- To run (execute) the program, type "a.out" or "test.fout" from the C Shell, depending upon which form of the command you used to compile your program.
Sometimes a single error in a program can cause further errors. You will not be able to execute the program correctly until these errors are fixed. Don't forget to recompile ("f77") your program after making changes. Otherwise, you'll be running an old and incorrect version when you type "a.out" or programname.fout (the filename you gave after the -o option).
There are Fortran-callable libraries such as the "NAG" and "IMSL" collections that greatly extend the power of Fortran. These libraries include routines for linear algebra, data analysis, statistics, special functions, etc. If you use any of these routines in your program, you must include additional information as the last options of the "f77" command. For NAG, add -L/opt/lib -lnag.
For IMSL, add $LINK_FNL $FFLAGS . In addition, for IMSL, type the
following commands (once) at the UNIX prompt (%) prior to your first compilation:
csh
source /opt/lib/ipt_1.2/ipt/bin/iptsetup.csh
And when you are completely done compiling and running your program, type
exit
at the UNIX prompt (%).
As an example, here is a complete program that reads in a number from the terminal and prints out the value of J0 (a Bessel function). The program uses
the NAG Fortran function, s17aef.
double precision x,y,s17aef
write(*,*) 'Enter a value for x'
read(*,*) x
y=s17aef(x,ier)
write(*,*) 'J0 at ', x, ' is ',y
end
If you create this source file with the name "J0.f", then the following UNIX commands will compile and run the program:
f77 -o J0.exe J0.f -L/opt/lib -lnag
J0.exe
Execution:
Enter a value for x
1.0
J0 at 1.0000000000000 = 0.76519768655797
Here is the same program calling the corresponding IMSL routine, dbsj0.
double precision x,y,dbsj0
write(*,*)'Enter a value for x'
read (*,*) x
y=dbsj0(x)
write(*,*) 'J0 at ',x,' = ',y
end
If you create this source file with the name "J0.f", then the followin
g UNIX commands will compile and run the program:
csh
source /opt/lib/ipt_1.2/ipt/bin/iptsetup.csh
f77 -o J0.exe J0.f $LINK_FNL $FFLAGS
J0.exe
Execution:
Enter a value for x
1.0
J0 at 1.0000000000000 = 0.76519768655797
Following are suggestions for creating and running programs in Scheme on UNIX.
Alternatively, you may want to use DrScheme instead, which is documented
elsewhere.
If you are a beginner or unfamiliar with UNIX, you should read the section "Writing Your Program." Using the "scm" impementation of Scheme involves alternating between editing your Scheme program (you must use vi as the editor) and running your program.
- Initiate a vi process and suspend it.
- If you are running an existing program stored in the file "fact.s", type
vi fact.s
and then suspend the process by typing "^Z". (The scheme program should have the same name as the file in which it's stored.)
- If you are writing a new program to be called, for example, "fact.s", type
vi fact.s
and type in the program using vi commands. The program might look like this:
(define (fact x)
(if (< x 2)
1
(* x (fact (- x 1)))
))
Use ":w" to save the program and then suspend the process by typing "^Z".
- Initiate the Scheme Program. (You do this only one time.)
Initiate the Scheme program by typing
scm
- Load and debug the Scheme program
- Load your program and run it as shown below. At the first Scheme prompt (>), load your program:
> (load "fact.s")
If there are no errors, you will again see the scheme prompt.
You can now test your function fact:
> (fact 3)
6
> (fact 5)
120
>
If you have errors, type "^Z" to suspend the Scheme process.
At this point you have 2 stopped jobs:
If you type the command "jobs", you get a listing of your stopped jobs:
"[1] - Stopped vi fact.s
[2] + Stopped scm"
To make corrections to your file, type
%v
This will put you back into vi. Correct your errors, type ":w" to save your changes, and type "^Z" to suspend vi. To get back into Scheme, type
%s
Type "^C" to get the Scheme prompt. Now load your program and repeat Step 3.
- Final Step
When you are finished, cancel both of your stopped jobs by typing
kill -9 %s %v
If you fail to do this and try to log out, the system will remind you that you have two stopped jobs. Type the "kill" command as shown above and then log out.

January 12, 1999